In lab 1, we have made our code work on a bare-metal computer (simulated by QEMU) successfully. However, it can do nothing but print some strings we hardcoded in the program on the terminal. Of course you can make it more complicated, such as factoring a large number, calculating the inverse of a matrix, etc. That’s cool but there are two significant drawbacks of this approach:
- The CPU runs a single program each time. Since the computing resources are precious(especially in the old time when you don’t have a modern OS), users who have many programs to run have to wait in front of the computer and manually load&start the next program after the previous one finished.
- Nobody wants to write the SBI and assembly level stuff every time, and it’s a duplication of efforts.
In order to solve these problems, people invented the Simple Batch Processing System
, which can load a batch of application programs and automatically execute them one by one. Besides, the Batch Processing System will provide some “library” code such as console output functions which may be reused by many programs.
A new problem arises when we use the batch process system: error handling. The user’s program may (often) run into errors, unconsciously or intentionally. We do not want the error of any program to affect others or the system, so the system should be able to handle these errors and terminate the programs when necessary. To achieve this goal we introduced the Privileges mechanism
and isolate user’s code from the system, which we will refer to as user mode
and kernel mode
. Note that this mechanism requires some support from hardware, and we will illustrate that with code in the following parts.
0x00 Privileges mechanism
The underlying reason for implementing the privileges mechanism is the system cannot trust any submitted program. Any errors or attacks could happen and may corrupt the system. We have to restrict users’ programs in an isolated “harmless” environment, where they have no access to 1) arbitrary memory or 2) any over-powerful instructions which may break the computer. In this lab, we mainly focus on the last point.
Prohibiting users’ program from using privileged instructions need the help from CPU. In riscv64, 4 levels of privileges are designed:
Level |
Encode |
Name |
0 |
00 |
U, User/Application |
1 |
01 |
S, Supervisor |
2 |
10 |
H, Hypervisor |
3 |
11 |
M, Machine |
All modes, except Machine
, have to go through binary interfaces provided by higher levels to control the hardware. The privileges level and their relation in our scenario are shown in the following figure:
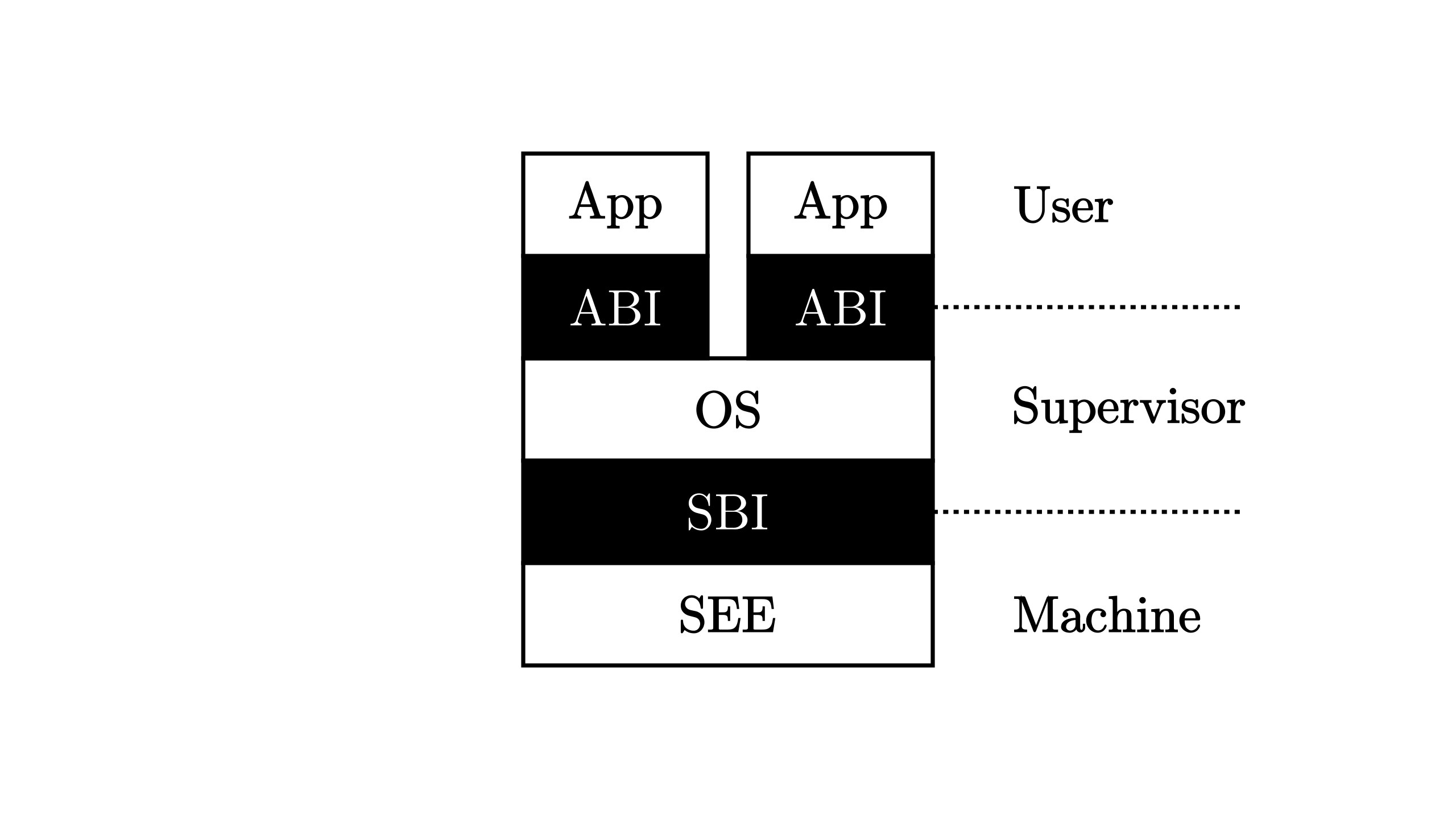
The binary interfaces between User mode and Supervisor mode are named Application Binary Interface (ABI), or another more famous one: syscall
.
继续阅读“rCore-OS Lab2: Batch Processing and Privileges”